일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | |||||
3 | 4 | 5 | 6 | 7 | 8 | 9 |
10 | 11 | 12 | 13 | 14 | 15 | 16 |
17 | 18 | 19 | 20 | 21 | 22 | 23 |
24 | 25 | 26 | 27 | 28 | 29 | 30 |
- 스택
- 딥러닝
- BAEKJOON
- loss
- machine learning
- DynamicProgramming
- pytorch
- MSE
- 홍콩과기대김성훈교수
- 백준
- Hypothesis
- 자연어처리
- Natural Language Processing with PyTorch
- 머신러닝 기초
- rnn
- Deep learning
- Softmax
- 파이썬
- tensorflow
- 강의자료
- 강의정리
- 머신러닝
- 정렬
- classifier
- 파이토치
- DP
- Cross entropy
- AI
- 알고리즘
- Python
- Today
- Total
개발자의시작
[Pytorch] 01-1 Tensor Manipulation 1 본문
글은 모두를위한딥러닝 시즌2 https://github.com/deeplearningzerotoall/PyTorch 을 정리한 글입니다.
GitHub - deeplearningzerotoall/PyTorch: Deep Learning Zero to All - Pytorch
Deep Learning Zero to All - Pytorch. Contribute to deeplearningzerotoall/PyTorch development by creating an account on GitHub.
github.com
앞으로 다룰 가장 기본적인 단위인 벡터(Vector), 매트릭스(Matrix), 텐서(Tensor)에 살펴본다.
위의 그림에는 없지만, 차원이 없는 값을 스칼라(Scalar)라고 한다. 첫 번째에 있는 1차원의 경우 벡터라고 한다. 두 번째 2차원으로 이루어진 경우 행렬, 즉 매트릭스에 해당한다. 3차원부터는 텐서(Tensor)라고 부르며, 4차원 이상의 경우 실제 세상과 차이가 있기 때문에 상상하기 어렵다. 쉽게 이해하기 위해 3차원의 텐서를 각각 위로 또는 옆으로 확장한 개념이라 생각하면 쉽다.
앞으로 다룰 매트릭스 또는 텐서들에 대해서 크기 계산이 매우 중요하다. 매 연산마다 텐서 또는 매트릭스의 크기를 항상 생각해야 제대로 된 구현을 할 수 있고, 새로운 코드를 접할 때도 쉽게 이해할 수 있다.
PyTorch Tensor
매트릭스 또는 텐서를 표현하는데, 위의 그림은 가장 기본적인 형태의 2차원 텐서이다.
텐서 |t|는 일반적으로 배치사이즈(batch_size), 와 차원(dim)을 갖는다. 즉, 배치사이즈 곱하기 차원의 사이즈를 갖는다고 볼 수 있다. 배치사이즈와 차원의 경우 보통의 경우 64차원, 256차원 등 2의 배수를 사용하는 것이 딥러닝 프로그램의 일반적인 방식이다.
더 나아가서 다른 영역의 딥러닝을 하게되면(ex. computer vision), 더 복잡한 형태의 입력과 출력을 사용한다. 이미지 처리를 예로 들면, 하나의 이미지는 가로와 세로로 이루어져 있고, 배치사이즈를 통해 여러 장의 이미지를 텐서로 표현할 수 있다.
텐서 |t|는 배치사이즈와 넓이(width) 그리고 높이를(heigth) 갖는다. 이와 같은 형태를 하나의 컨벤션(convention)이라 하며, 위의 그림과 다른 형태로 표현할 수도 있다.
이전에는 비전에 대해 다루었는데, 시계열 데이터나 순차 정보가 있는 시퀀스 데이터를 다루는 자연어처리(nlp)의 경우에도 3차원 텐서를 가지고 일반적인 입력과 출력을 다룰 수 있다.
텐서 |t|는 배치사이즈 길이(length) 그리고 차원(dim)을 갖는다. 비전도 마찬가지이며, 하나의 배치사이즈 내에 있는 텐서는 하나의 문장이 되며, 배치의 방향으로 쌓여 있다고 생각할 수 있다.
본격적으로, 파이토치를 활용하기에 앞서 numpy에 대해 설명한다.
Run pip install -r requirements.txt in terminal to install all required Python packages.
1D Array with NumPy
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
import numpy as np
import torch
t = np.array([0., 1., 2., 3., 4., 5., 6.])
print(t)
print("Rank of t: ", t.ndim)
print("Shape of t: ", t.shape)
print("t[0] t[1] t[-1] =", t[0], t[1], t[-1])
print("t[2:5] t[4:-1]", t[2:5], t[4:-1])
print("t[:2] t[3:] =", t[:2], t[3:])
|
2D Array with NumPy
1
2
3
4
5
6
|
t= np.array([[1., 2., 3.], [4., 5., 6.], [7., 8., 9.], [10., 11., 12.]])
print(t)
print("Rank of t : ", t.ndim)
print("Shape of t : ", t.shape)
|
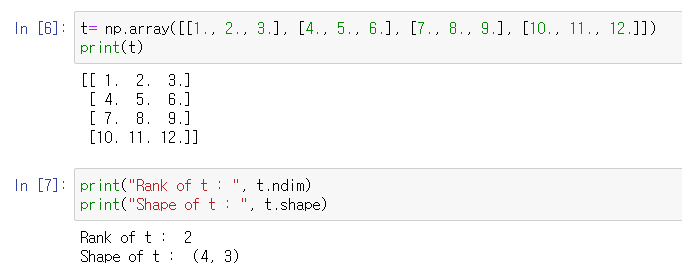
다음은 PyTorch Tensor에 대해 다룬다.
PyTorch Tensor
1
2
3
4
5
6
7
8
9
|
t= torch.FloatTensor([0., 1., 2., 3., 4., 5., 6.])
print(t)
print(t.dim())
print(t.shape)
print(t.size())
print(t[0], t[1], t[-1])
print(t[2:5], t[4:-1])
print(t[0:2], t[3:] )
|
1
2
3
4
5
6
7
8
9
10
11
12
|
t= torch.FloatTensor([[1., 2., 3.],
[4., 5., 6.],
[7., 8., 9.],
[10., 11., 12.]
])
print(t)
print(t.dim())
print(t.size())
print(t[:, 1])
print(t[:, 1].size())
print(t[:, :-1])
|
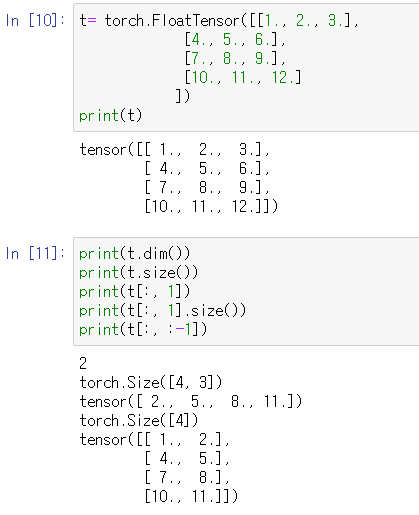
Broadcasting
Broadcasting : Tensor의 크기를 자동으로 맞춰 주는 것.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
m1= torch.FloatTensor([[3,3,]])
m2= torch.FloatTensor([[2,2,]])
print(m1 + m2)
#Vector + Scalar
m1 = torch.FloatTensor([[1,2]])
m2 = torch.FloatTensor([3])
print(m1 + m2)
# 2 x 1 Vector + 1 x 2 Vector
m1 = torch.FloatTensor([[1,2,]])
m2 = torch.FloatTensor([[3], [4]])
print(m1 + m2)
|
Pytorch로 프로그래밍시 Broadcasting은 매우 편리한 기능이지만, 잘못된 데이터 형식이나 데이터 입력이 주어져도 에러가 발생하지 않고 프로그램이 동작하기 때문에 주의해야 한다.
Multiplication vs Matrix Multiplication
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
print()
print("----------")
print("Mul vs Matmul")
print("----------")
m1 = torch.FloatTensor([[1, 2], [3, 4]])
m2 = torch.FloatTensor([[1], [2]])
print("Shape of Matrix 1:", m1.shape) # 2 x 2
print("Shape of Matrix 2:", m1.shape) # 2 x 1
print(m1.matmul(m2))
m1 = torch.FloatTensor([[1, 2], [3, 4]])
m2 = torch.FloatTensor([[1], [2]])
print("Shape of Matrix 1:", m1.shape) # 2 x 2
print("Shape of Matrix 2:", m1.shape) # 2 x 1
print(m1 * m2)
print(m1.mul(m2))
|
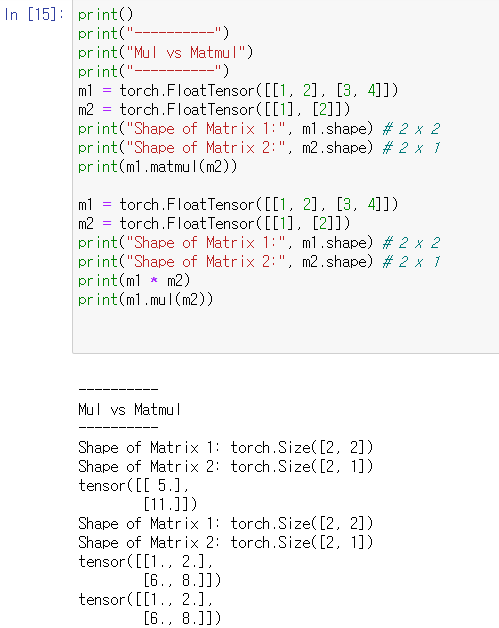
Mean
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
t = torch.FloatTensor([1, 2])
print(t.mean())
#Can't use mean() on integers
t= torch.LongTensor([1, 2])
try:
print(t.mean())
except Exception as exc:
print(exc)
t = torch.FloatTensor([[1, 2], [3, 4]])
print(t)
print(t.mean())
print(t.mean(dim=0))
print(t.mean(dim=1))
print(t.mean(dim=-1))
|
Sum
1
2
3
4
5
6
7
|
t = torch.FloatTensor([[1, 2], [3, 4]])
print(t)
print(t.sum())
print(t.sum(dim=0))
print(t.sum(dim=1))
print(t.sum(dim=-1))
|
Max and Argmax
※Argmax 는 배열 내 가장 큰 값이 있는 위치(인덱스, index)의 값.
1
2
3
4
5
6
7
8
9
10
11
|
t = torch.FloatTensor([[1, 2], [3, 4]])
print(t)
print(t.max())
print(t.max(dim=0))
print("Max: ", t.max(dim=0)[0])
print("Argmax: ", t.max(dim=0)[1])
print(t.max(dim=1))
print(t.max(dim=-1))
|
'머신러닝(machine learning)' 카테고리의 다른 글
[Pytorch] 02 Linear regression (0) | 2021.11.30 |
---|---|
[Pytorch] 01-2 Tensor Manipulation 2 (0) | 2021.11.26 |
도커[Docker] Instruction (0) | 2021.11.18 |
[머신러닝][딥러닝] Linear Regression의 cost 최소화 minimize cost 3.0 (0) | 2020.04.23 |
[머신러닝][딥러닝] TensorFlow로 간단한 linear regression 구현 2.1 (0) | 2020.04.08 |