일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | |||||
3 | 4 | 5 | 6 | 7 | 8 | 9 |
10 | 11 | 12 | 13 | 14 | 15 | 16 |
17 | 18 | 19 | 20 | 21 | 22 | 23 |
24 | 25 | 26 | 27 | 28 | 29 | 30 |
- 알고리즘
- Softmax
- 강의정리
- Deep learning
- MSE
- classifier
- 강의자료
- 머신러닝 기초
- rnn
- tensorflow
- AI
- Cross entropy
- 자연어처리
- DP
- 스택
- 머신러닝
- 정렬
- 파이썬
- 딥러닝
- machine learning
- Python
- 백준
- loss
- BAEKJOON
- 홍콩과기대김성훈교수
- DynamicProgramming
- Natural Language Processing with PyTorch
- 파이토치
- pytorch
- Hypothesis
- Today
- Total
개발자의시작
[Pytorch] 01-2 Tensor Manipulation 2 본문
글은 모두를위한딥러닝 시즌2 https://github.com/deeplearningzerotoall/PyTorch 을 정리한 글입니다.
GitHub - deeplearningzerotoall/PyTorch: Deep Learning Zero to All - Pytorch
Deep Learning Zero to All - Pytorch. Contribute to deeplearningzerotoall/PyTorch development by creating an account on GitHub.
github.com
View (Reshape)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
import numpy as np
import torch
t = np.array([[[0, 1, 2],
[3, 4, 5]],
[[6, 7, 8],
[9, 10, 11]]])
ft = torch.FloatTensor(t)
print(ft.shape)
print(ft.view([-1, 3]))
print(ft.view([-1, 3]).shape)
print(ft.view([-1, 1, 3]))
print(ft.view([-1, 1, 3]).shape)
|
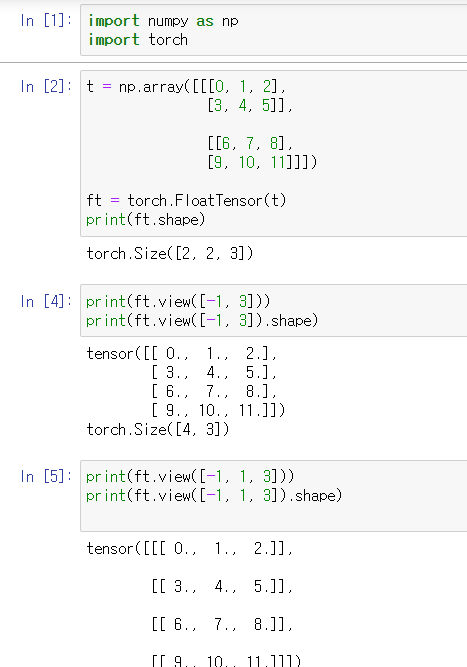
Squeeze
※ 해당 dim의 element의 개수가 1일 경우 해당 dim을 없애준다.
squeeze(dim = ? )과 같은 형태로 특정 차원을 지정하여 적용할 수 있다.
1
2
3
4
5
6
|
ft = torch.FloatTensor([[0], [1], [2]])
print(ft)
print(ft.shape)
print(ft.squeeze())
print(ft.squeeze().shape)
|
Unsqueeze
※ Unsqueeze: 원하는 dim의 차원을 1로 만들어준다. (squeeze와 다르게 반드시 원하는 차원을 지정해줘야 한다.
unsqueeze( dim= ?)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
ft = torch.Tensor([0, 1, 2])
print(ft.shape)
print(ft.unsqueeze(0))
print(ft.unsqueeze(0).shape)
print(ft.view(1, -1))
print(ft.view(1, -1).shape)
print(ft.unsqueeze(1))
print(ft.unsqueeze(1).shape)
print(ft.unsqueeze(-1))
print(ft.unsqueeze(-1).shape)
|
Type Casting
※ 텐서의 타입을 바꿔주는 것.
※ bt= ByteTensor
1
2
3
4
5
6
7
8
9
10
|
lt = torch.LongTensor([1, 2, 3, 4])
print(lt)
print(lt.float())
bt = torch.ByteTensor([True, False, False, True])
print(bt)
print(bt.long())
print(bt.flat())
|
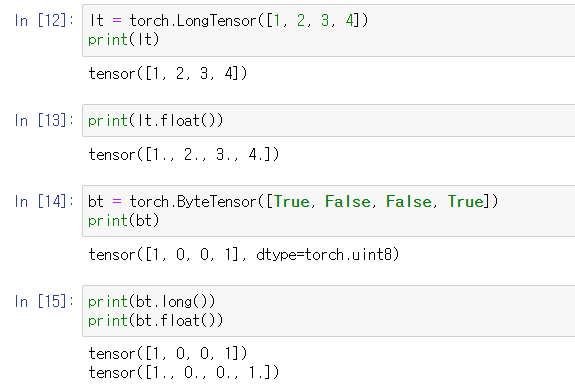
Concatenate
※ Concatenate : 두 텐서를 이어 붙이는 것.
1
2
3
4
|
x = torch.FloatTensor([[1, 2], [3, 4]])
y = torch.FloatTensor([[5, 6], [7, 8]])
print(torch.cat([x, y], dim=0))
print(torch.cat([x, y], dim=1))
|
Stacking
※ concatenate의 작업을 단축시켜 놓은 것 ( 3개 이상의 텐서를 쌓을 수 있다)
1
2
3
4
5
6
7
8
|
x = torch.FloatTensor([1, 4])
y = torch.FloatTensor([2, 5])
z = torch.FloatTensor([3, 6])
print(torch.stack([x, y, z]))
print(torch.stack([x, y, z], dim=1))
print(torch.cat([x.unsqueeze(0), y.unsqueeze(0), z.unsqueeze(0)], dim=0))
|
Ones and Zeros
※ 프로그래밍 시 cpu 텐서와 gpu 텐서를 동시에 더하거나 빼는 등의 연산을 수행하면 에러가 난다. 같은 디바이스의 텐서여야만 연산을 할 수 있다. 이런 경우 ones와 zeros를 사용하면 같은 디바이스의 텐서를 선언해준다.
1
2
3
4
5
|
x = torch.FloatTensor([[0, 1, 2], [2, 1, 0]])
print(x)
print(torch.ones_like(x))
print(torch.zeros_like(x))
|
In-place Operation
※ 같은 값을 곱하더라도 결과가 다르게 출력된다. In-place Operation은 연산 후 해당 값을 선언과 동시에 저장하여 새로운 텐서를 할당하지 않고도 연산을 수행할 수 있다. (Python의 경우 가비지 컬렉터가 효율적으로 설계돼 있어서 크게 신경 쓰지 않아도 된다.)
1
2
3
4
5
6
|
x = torch.FloatTensor([[1, 2], [3, 4]])
print(x.mul(2.))
print(x)
print(x.mul_(2.))
print(x)
|
'머신러닝(machine learning)' 카테고리의 다른 글
[Pytorch] 03 Deeper Look at GD (0) | 2021.11.30 |
---|---|
[Pytorch] 02 Linear regression (0) | 2021.11.30 |
[Pytorch] 01-1 Tensor Manipulation 1 (0) | 2021.11.26 |
도커[Docker] Instruction (0) | 2021.11.18 |
[머신러닝][딥러닝] Linear Regression의 cost 최소화 minimize cost 3.0 (0) | 2020.04.23 |